Expectation-Maximization (EM) Algorithm
The Expectation-Maximization (EM) algorithm is a powerful iterative method used in statistical computing for finding maximum likelihood estimates in the presence of latent variables. This algorithm is widely applied in various domains, including machine learning, data clustering, natural language processing, and computational biology. Due to its robustness in handling incomplete data, the EM algorithm has become an indispensable tool in probabilistic modeling.
Table of Contents
- Understanding the EM Algorithm
- Maximum Likelihood Estimation (MLE)
- Handling Missing Data
- Key Steps in the EM Algorithm
- Mathematical Formulation
- Convergence Properties and Challenges
- Applications of the EM Algorithm
- Significance of the EM Algorithm
- Implementation of the EM Algorithm in Python
- Conclusion
Understanding the EM Algorithm
The Expectation-Maximization (EM) algorithm is a widely used approach in statistical estimation problems involving incomplete or missing data. It is especially useful when direct computation of maximum likelihood estimates (MLE) is challenging due to latent variables.
Maximum Likelihood Estimation (MLE)
Maximum Likelihood Estimation (MLE) is a fundamental statistical technique for estimating the parameters of a probability distribution by maximizing the likelihood function. Given a set of observed data points X = {x1,x2,….,xn} and a probability distribution parameterized by θ, the likelihood function is defined as:
L(θ|X) = P(X|θ)
The goal of MLE is to find the parameter θ that maximizes this likelihood function:
= argθ max L(θ|X)
However, in cases where some variables are unobserved or latent, the likelihood function becomes intractable. The EM algorithm provides an iterative solution to this problem.
Handling Missing Data
In many real-world scenarios, datasets often contain missing values due to factors like measurement errors, data corruption, or privacy concerns. Direct estimation using traditional methods becomes difficult because the missing values obscure the structure of the data distribution.
The EM algorithm overcomes this limitation by treating the missing data as latent variables and iteratively estimating them to refine parameter estimates. It does so by computing the expected values of the missing variables and using those expectations to maximize the likelihood function.
Key Steps in the EM Algorithm
The EM algorithm follows two primary iterative steps:
1. Expectation Step (E-step):
In this step, we compute the expected value of the complete-data log-likelihood function by integrating over the possible values of the missing data given the observed data and current estimates of the parameters. Mathematically, the expectation step is represented as:
Q(θ|θ(t)) = Ez|X,θ(t)[logP(X,Z|θ)]
where:
- Z represents the missing or latent variables,
- θ(t) is the current estimate of the parameters,
- Q(θ|θ(t)) is the expected log-likelihood function.
This step essentially replaces the missing data with its expected value based on current parameter estimates.
2. Maximization Step (M-step):
In the M-step, we maximize the expected log-likelihood function obtained from the E-step to update the parameter estimates. The new estimate of θ is obtained as:
θ(t+1) = argθ max Q(θ|θ(t))
This step finds the parameter values that maximize the likelihood function using the expected values computed in the E-step.
3. Iteration Until Convergence:
The E-step and M-step are repeated iteratively until the parameter estimates converge, meaning that the changes in parameter values between iterations become negligible.
Mathematical Formulation
To better understand the EM algorithm, let’s consider a general probability model with observed data X , missing data Z , and parameters θ. The marginal likelihood of the observed data is:
P(X|θ) =
Since direct maximization of this likelihood function is often difficult, we introduce the concept of the complete-data log-likelihood:
log
The EM algorithm constructs an auxiliary function Q(θ|θ(t)) that serves as a surrogate for the intractable log-likelihood function by taking the expectation over the missing variables. This auxiliary function is maximized in the M-step to iteratively refine the parameter estimates.
Convergence Properties and Challenges
The EM algorithm is guaranteed to produce a sequence of parameter estimates that never decrease the likelihood function. However, there are some important considerations:
- Monotonic Convergence: Each iteration increases (or maintains) the likelihood function value, ensuring stable convergence.
- Local Optima: Since the EM algorithm uses iterative updates, it may converge to a local maximum rather than the global optimum. To mitigate this, multiple initializations are often used.
- Slow Convergence: The rate of convergence can be slow, particularly when the missing data significantly affects the likelihood function.
- Computational Complexity: While the algorithm is efficient for small to moderate datasets, the computational cost can become prohibitive for very large datasets with high-dimensional latent variables.
Despite these challenges, the EM algorithm remains a cornerstone of probabilistic inference and unsupervised learning.
Applications of the EM Algorithm
The Expectation-Maximization (EM) algorithm has a wide range of applications across various fields, thanks to its capability of handling missing or latent data. Some key applications include:
1. Clustering in Machine Learning
- The EM algorithm is widely used in probabilistic clustering methods, such as Gaussian Mixture Models (GMMs).
- Unlike k-means, which uses hard clustering, GMM assigns probabilistic cluster memberships, making it useful in soft clustering applications.
2. Natural Language Processing (NLP)
- Used in applications like word sense disambiguation, machine translation, and text classification.
- It helps in modeling hidden structures in textual data, such as latent topic models in Latent Dirichlet Allocation (LDA).
3. Computer Vision
- EM is used in image segmentation, object recognition, and motion tracking.
- For example, in image segmentation, it helps in identifying different regions based on probabilistic models.
4. Bioinformatics & Genetics
- Used in gene sequence analysis, haplotype inference, and protein structure prediction.
- It helps in estimating missing genetic markers and modeling biological sequences.
5. Econometrics and Finance
- EM is employed in credit scoring, risk assessment, and time-series forecasting.
- It is useful in estimating hidden states in financial models such as Hidden Markov Models (HMMs).
6. Medical Data Analysis
- EM plays a role in medical image reconstruction, disease diagnosis, and patient clustering.
- It is used in MRI image processing to improve clarity and detect abnormalities.
7. Speech Recognition
- In Hidden Markov Models (HMMs), the EM algorithm helps in estimating transition probabilities.
- It is widely used in automatic speech recognition (ASR) systems.
These applications highlight the adaptability of the EM algorithm across multiple domains where missing or latent data needs to be estimated.
Significance of the EM Algorithm
The EM algorithm holds significant importance in statistical estimation due to its robustness and adaptability. Here are some of the key reasons why it is widely used:
1. Handles Missing Data Effectively
- One of the biggest advantages of the EM algorithm is its ability to estimate missing or unobserved data, making it highly useful in real-world datasets that are often incomplete.
2. Probabilistic Clustering
- Unlike deterministic clustering methods, EM allows soft clustering, where data points can belong to multiple clusters with different probabilities. This is crucial in applications like GMM-based clustering.
3. Improves Parameter Estimation
- EM provides an iterative method to refine parameter estimates and maximize likelihood, leading to improved performance in machine learning models.
4. Applicable to a Wide Range of Models
- It is used in Gaussian Mixture Models (GMMs), Hidden Markov Models (HMMs), and Bayesian Networks, making it a fundamental algorithm in probabilistic modeling.
5. Mathematically Guaranteed to Converge
- The likelihood function increases in each iteration, ensuring convergence (though it may be to a local optimum).
6. Used in High-Dimensional Data Analysis
- EM is particularly beneficial when dealing with high-dimensional datasets where direct optimization methods are computationally infeasible.
Despite these advantages, the EM algorithm also has some limitations, such as slow convergence and susceptibility to local optima. However, it remains a powerful tool for statistical estimation and inference.
Implementation of the EM Algorithm
import numpy as np
from scipy.stats import norm
# Generate synthetic data
np.random.seed(42)
data = np.concatenate([np.random.normal(5, 1, 100), np.random.normal(10, 1, 100)])
# Initialize parameters
mu1, mu2 = 4, 9
sigma1, sigma2 = 1, 1
pi = 0.5
for _ in range(100):
# E-step
resp1 = pi * norm.pdf(data, mu1, sigma1)
resp2 = (1 - pi) * norm.pdf(data, mu2, sigma2)
gamma = resp1 / (resp1 + resp2)
# M-step
mu1 = np.sum(gamma * data) / np.sum(gamma)
mu2 = np.sum((1 - gamma) * data) / np.sum(1 - gamma)
pi = np.mean(gamma)
print(f'Estimated Means: {mu1}, {mu2}')
Conclusion
The Expectation-Maximization (EM) algorithm is a versatile and powerful statistical technique for parameter estimation in the presence of missing data. It is widely used in various domains, including clustering, machine learning, and bioinformatics. While the algorithm guarantees convergence, it may suffer from local optima and slow convergence in certain cases. Nevertheless, its ability to handle incomplete data and provide stable parameter estimates makes it a fundamental tool in probabilistic modeling. Understanding and implementing the EM algorithm enables statisticians and data scientists to solve complex problems where direct likelihood maximization is not feasible. By leveraging its iterative nature, we can improve parameter estimates and make meaningful inferences from incomplete datasets.
Featured Blogs
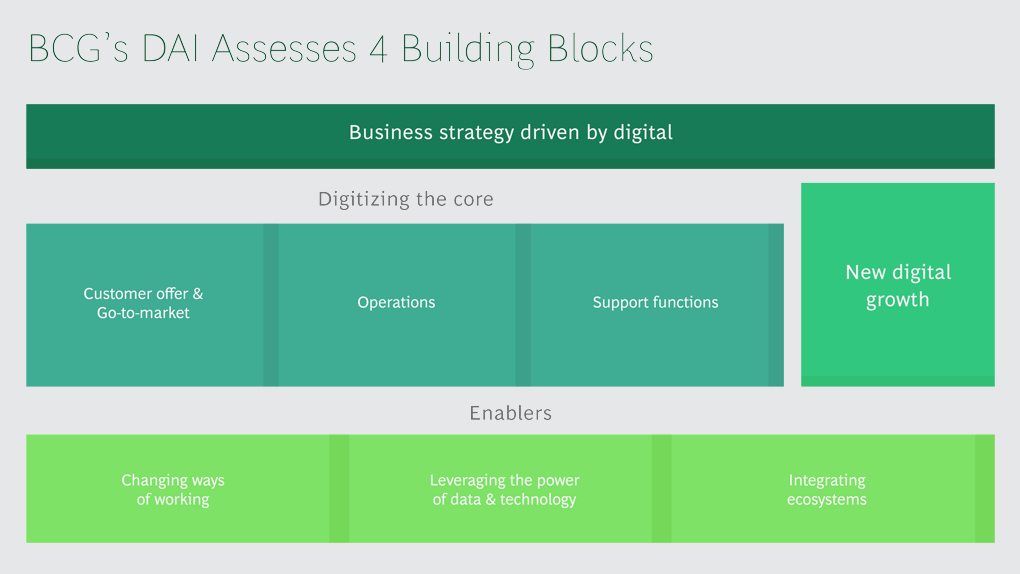
BCG Digital Acceleration Index
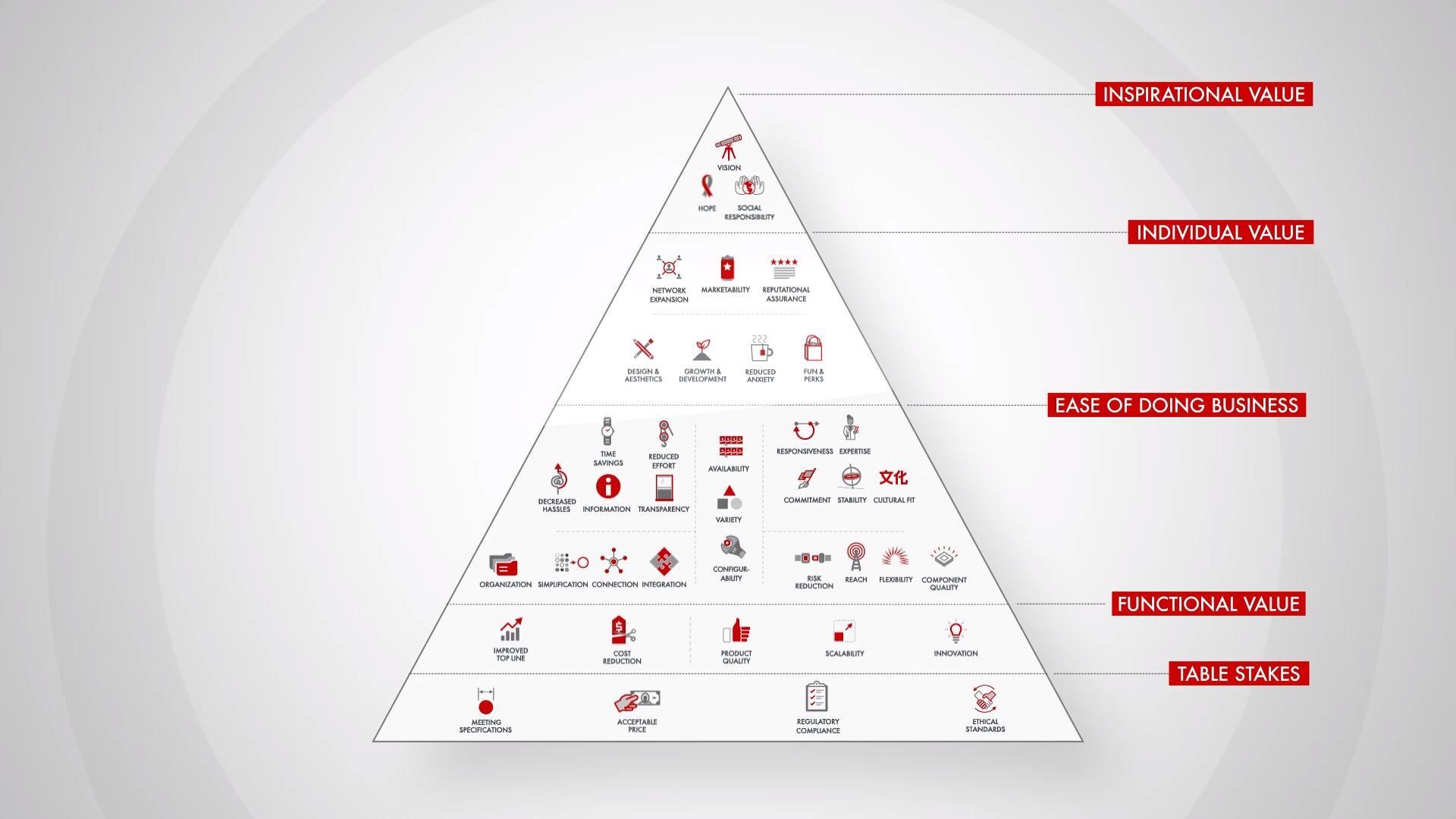
Bain’s Elements of Value Framework
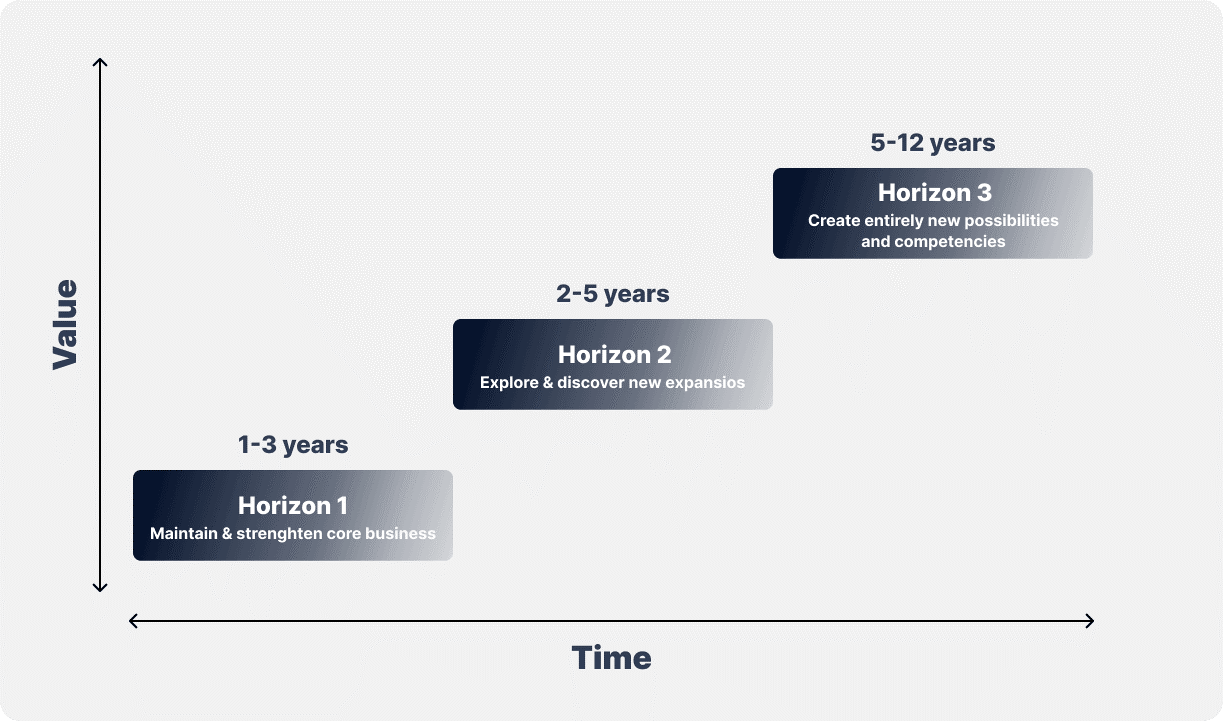
McKinsey Growth Pyramid
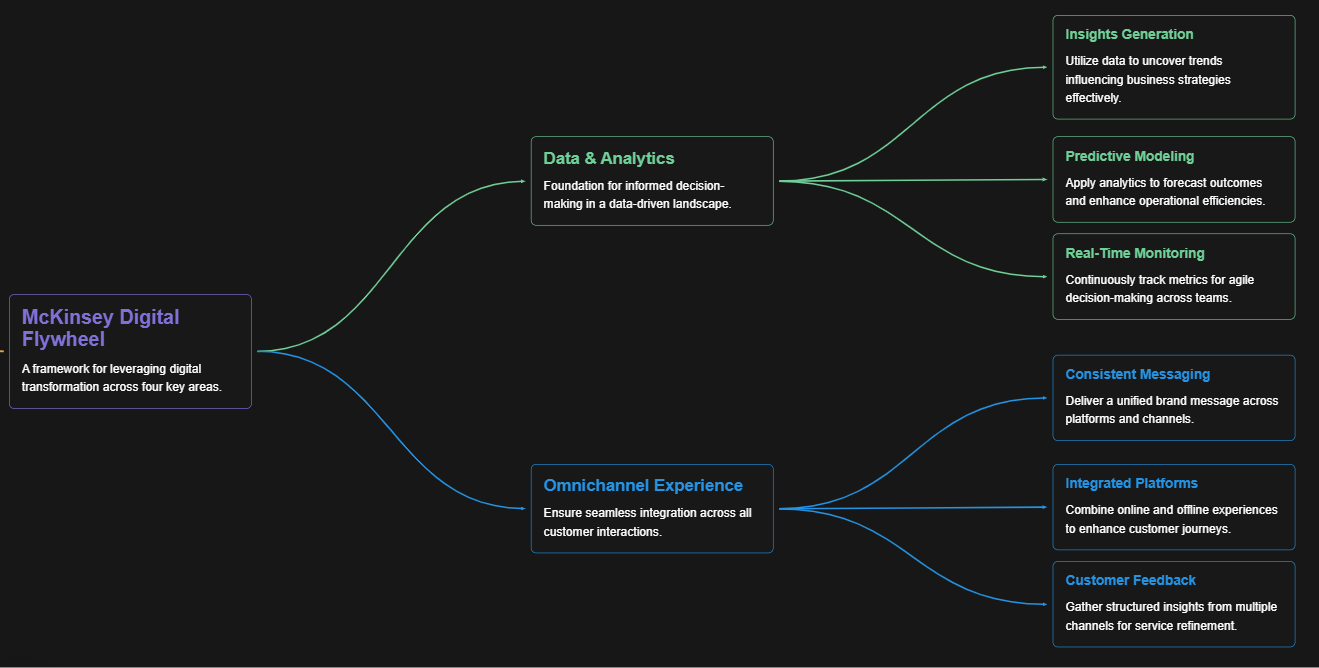
McKinsey Digital Flywheel
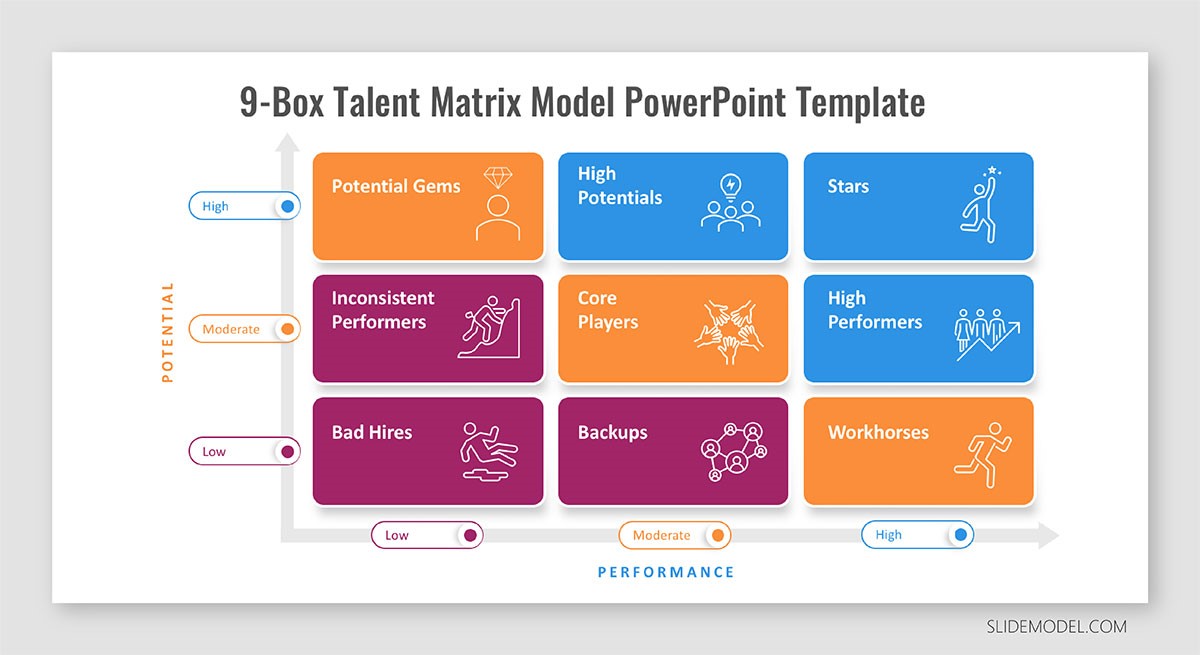
McKinsey 9-Box Talent Matrix
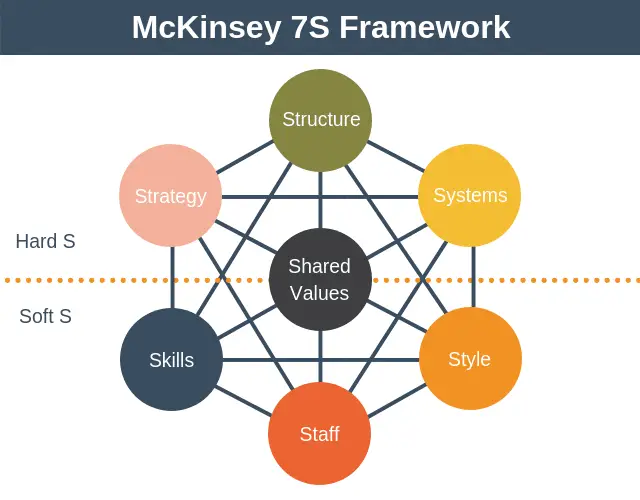
McKinsey 7S Framework
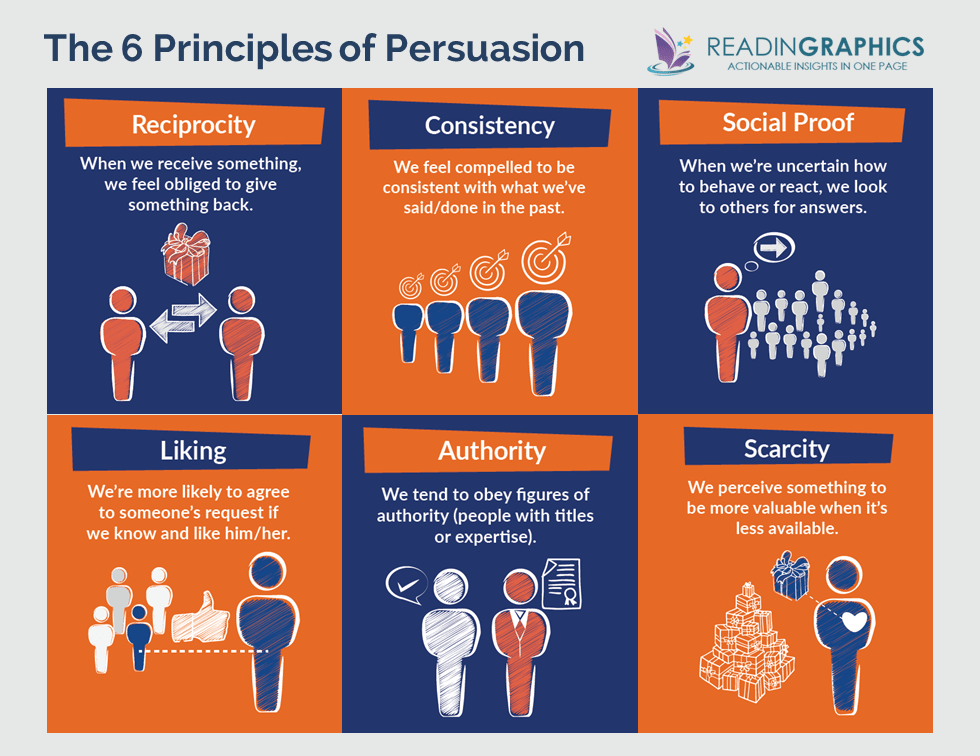
The Psychology of Persuasion in Marketing
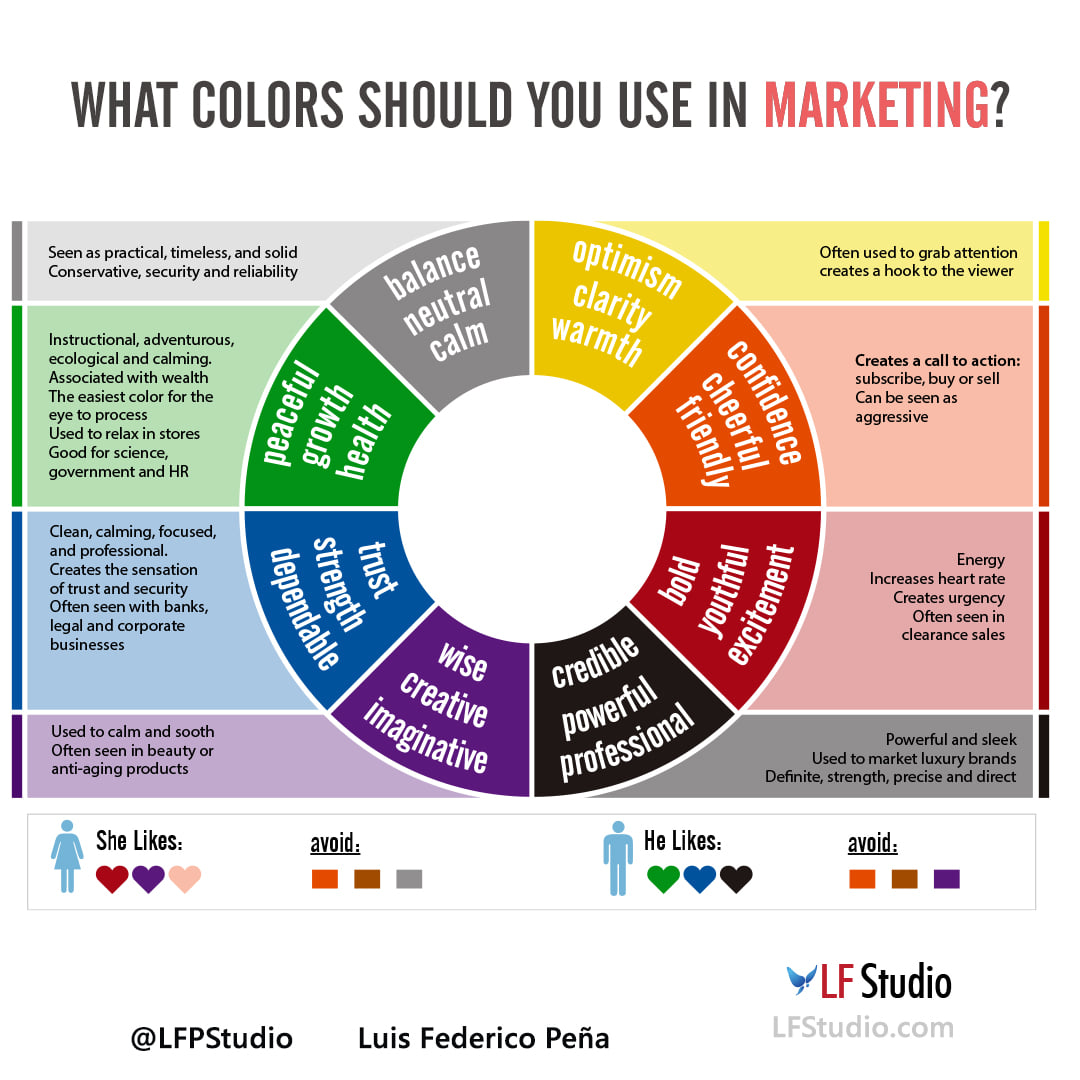
The Influence of Colors on Branding and Marketing Psychology
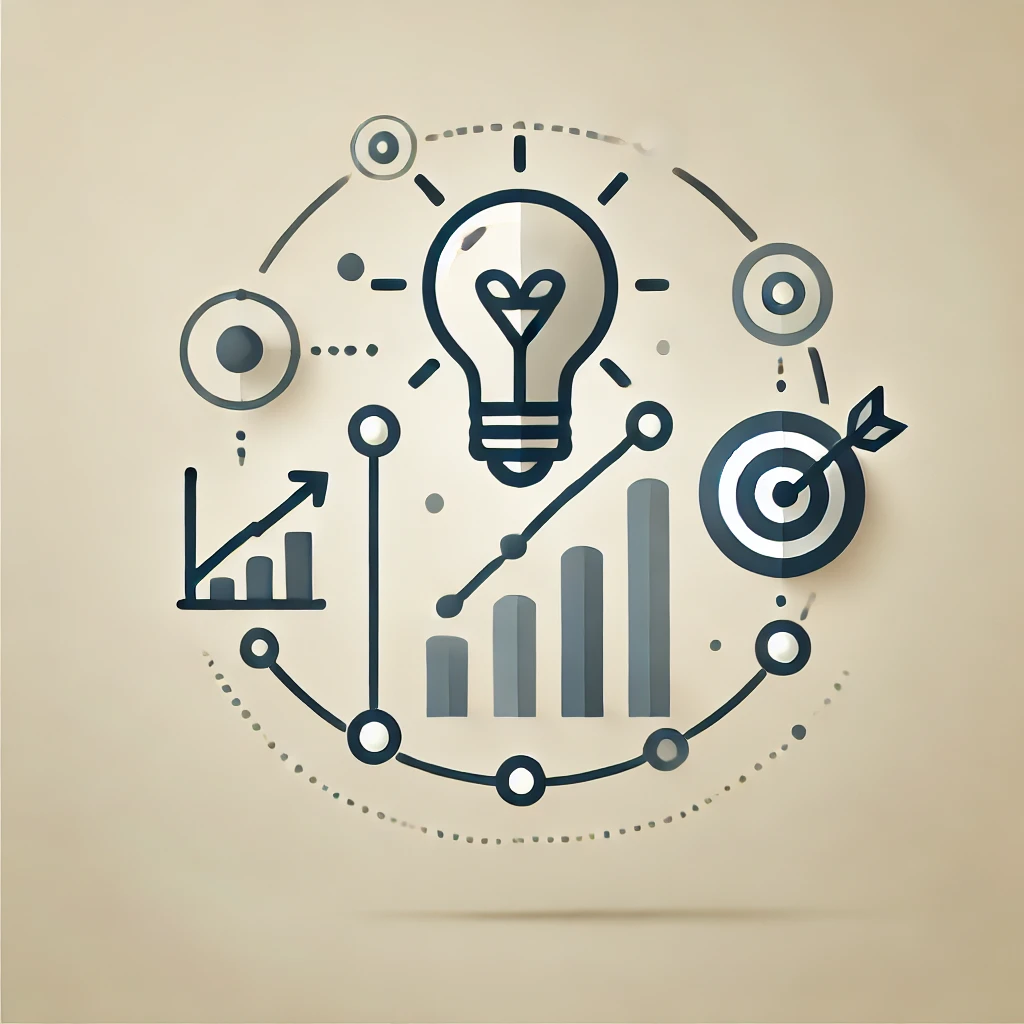
What is Marketing?
Recent Blogs
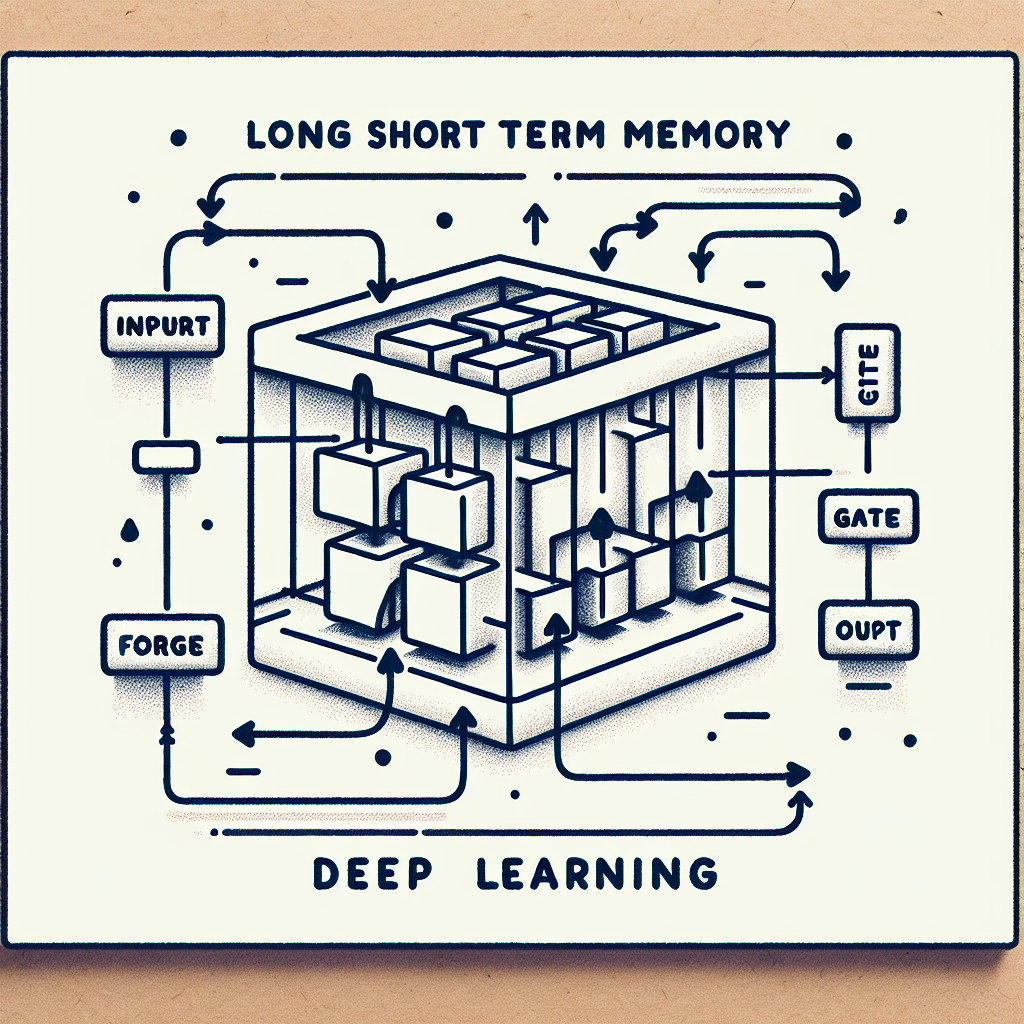
Part 2: The Gatekeeper – Long Short-Term Memory (LSTM) Networks
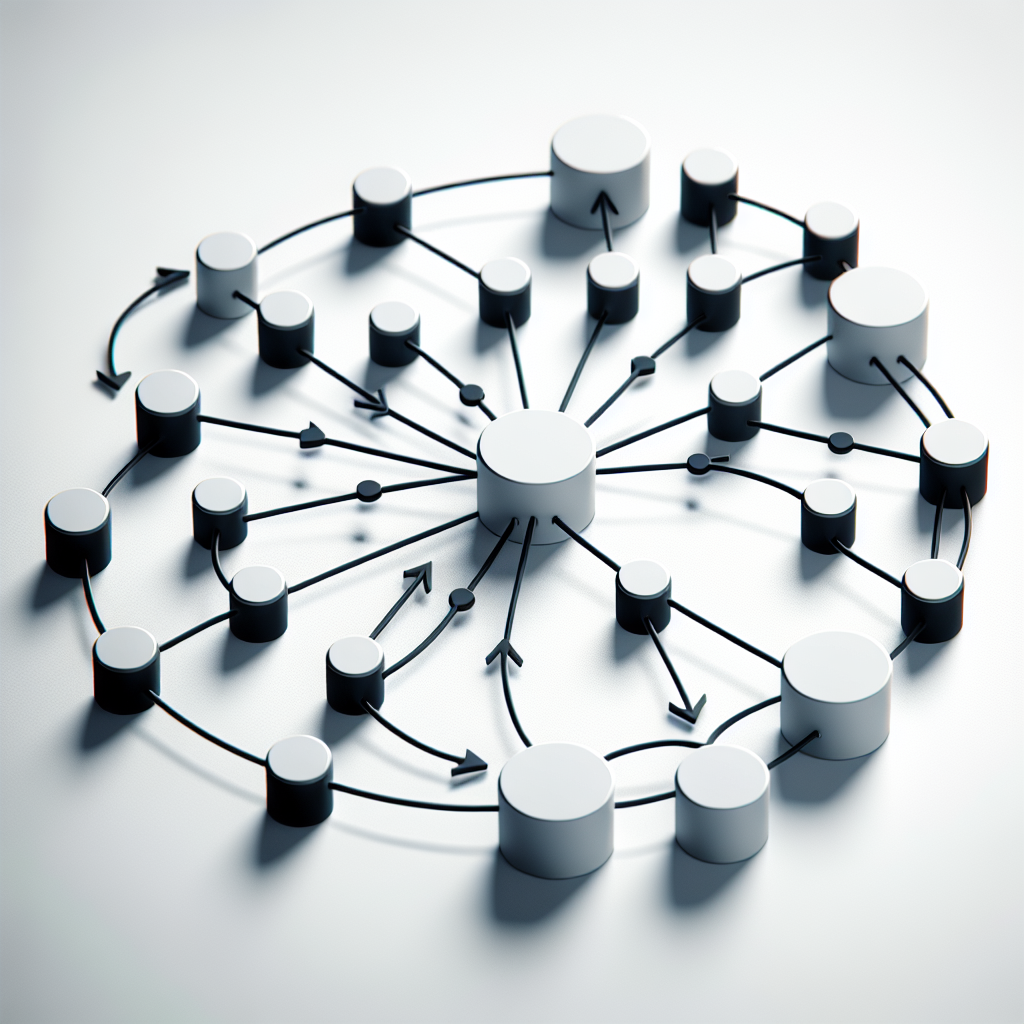
Part 1: The Roots – Recurrent Neural Networks (RNNs)
How Hierarchical Priors Helped Anita Make Smarter, Faster Marketing Decisions
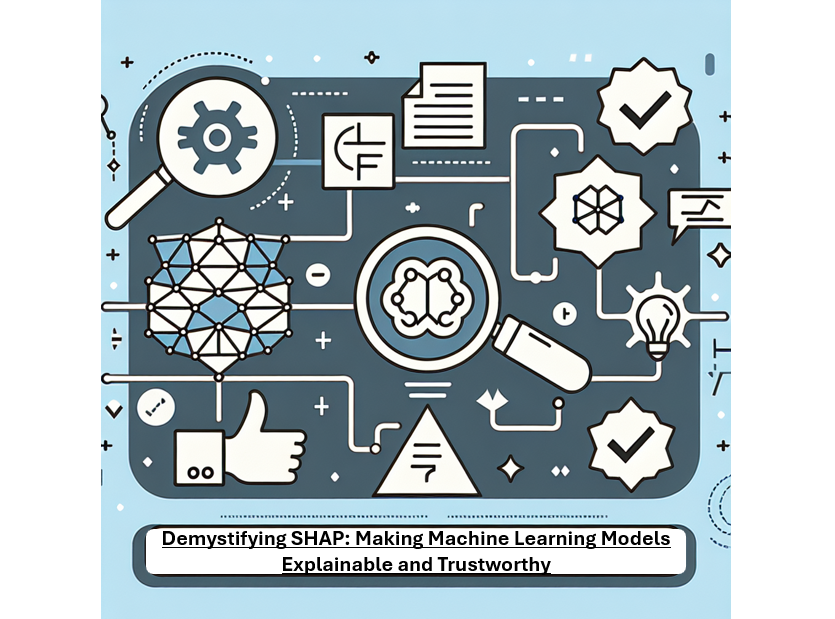
Demystifying SHAP: Making Machine Learning Models Explainable and Trustworthy
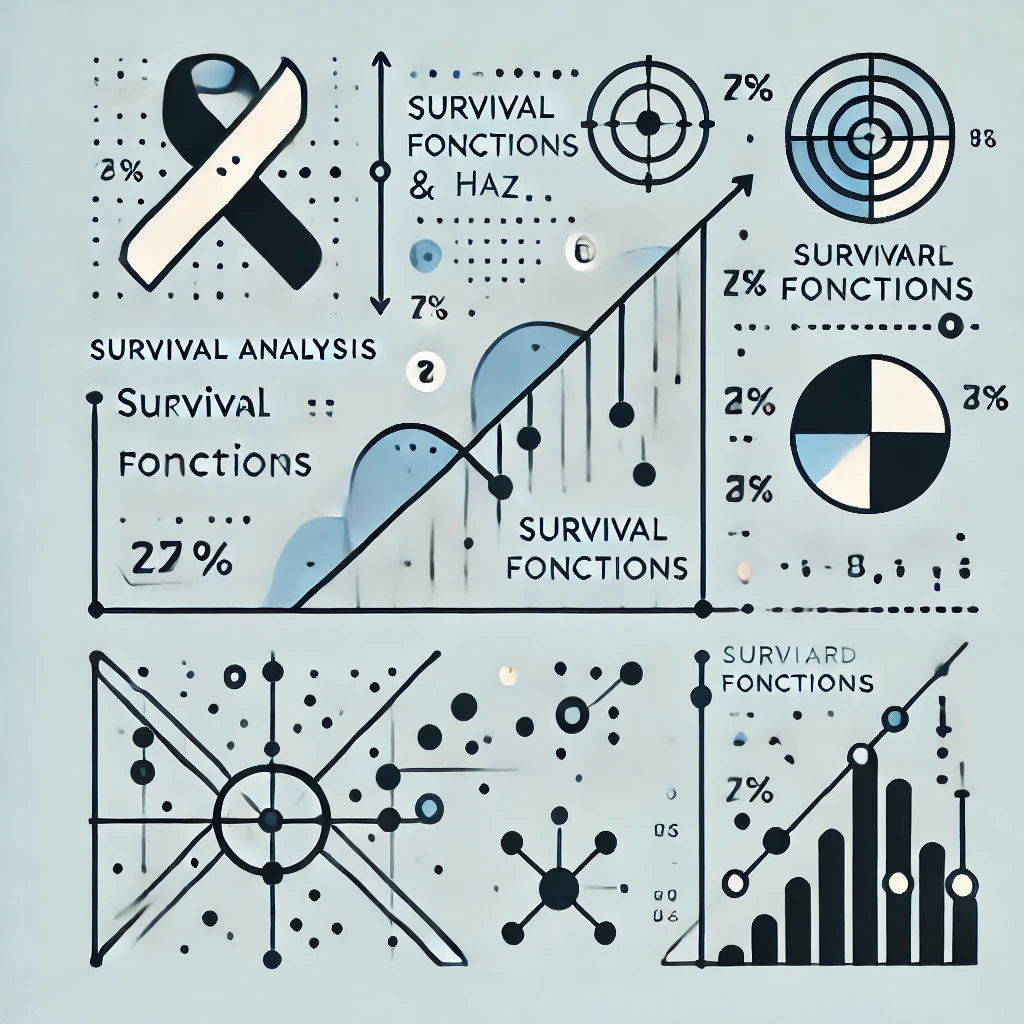
Survival Analysis & Hazard Functions: Concepts & Python Implementation
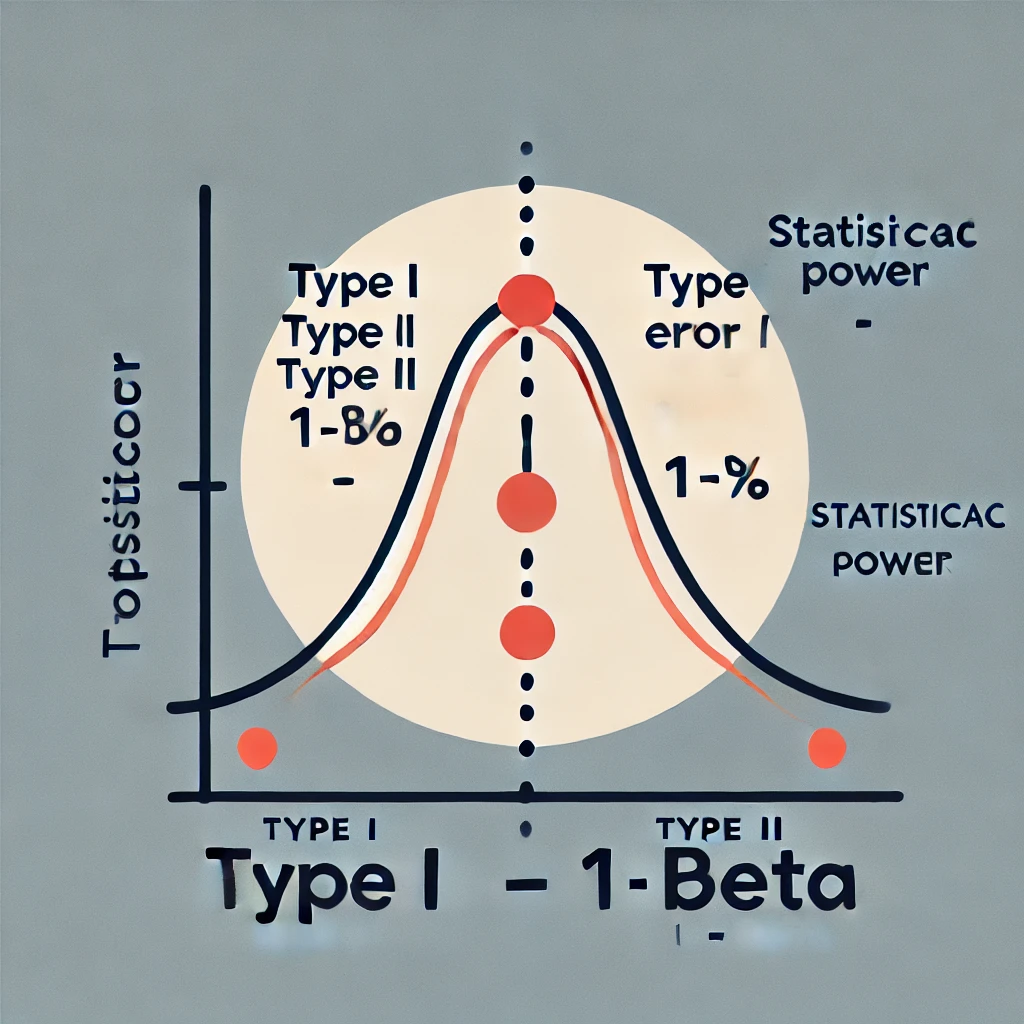